Mastering Divide and Conquer Algorithms for Efficient Problem Solving
Divide and conquer algorithms stand as a cornerstone of computer science, utilizing a blend of algorithmic prowess and systematic logic to break down complex problems into more manageable parts. From sorting lists with ease to automating intricate tasks, these algorithms operate on the principles of a logarithm, ensuring that time complexity remains at bay. Renowned for their efficiency, divide and conquer strategies power numerous search algorithms, tirelessly working behind the scenes like an invisible equator, allocating resources and simplifying tasks. For those eager to hone their coding skills and amplify their programming toolkit, the journey into the realm of divide and conquer algorithms offers a path to sophistication and speed. Keep reading to uncover the layered intricacies and applications of these powerful algorithms that are reshaping problem-solving in the digital age.
Key Takeaways
- Divide and Conquer Algorithms Simplify Complex Problems for Efficient Computation
- Recursion Is a Core Principle in Divide and Conquer, Enhancing Problem-Solving Capabilities
- Big O Notation Plays a Crucial Role in Evaluating Algorithm Efficiency and Performance
- Merge Sort and Quick Sort Demonstrate the Effectiveness of Divide and Conquer in Sorting
- Understanding When to Use Divide and Conquer Versus Dynamic Programming Is Key for Optimization
How Divide and Conquer Algorithms Work?
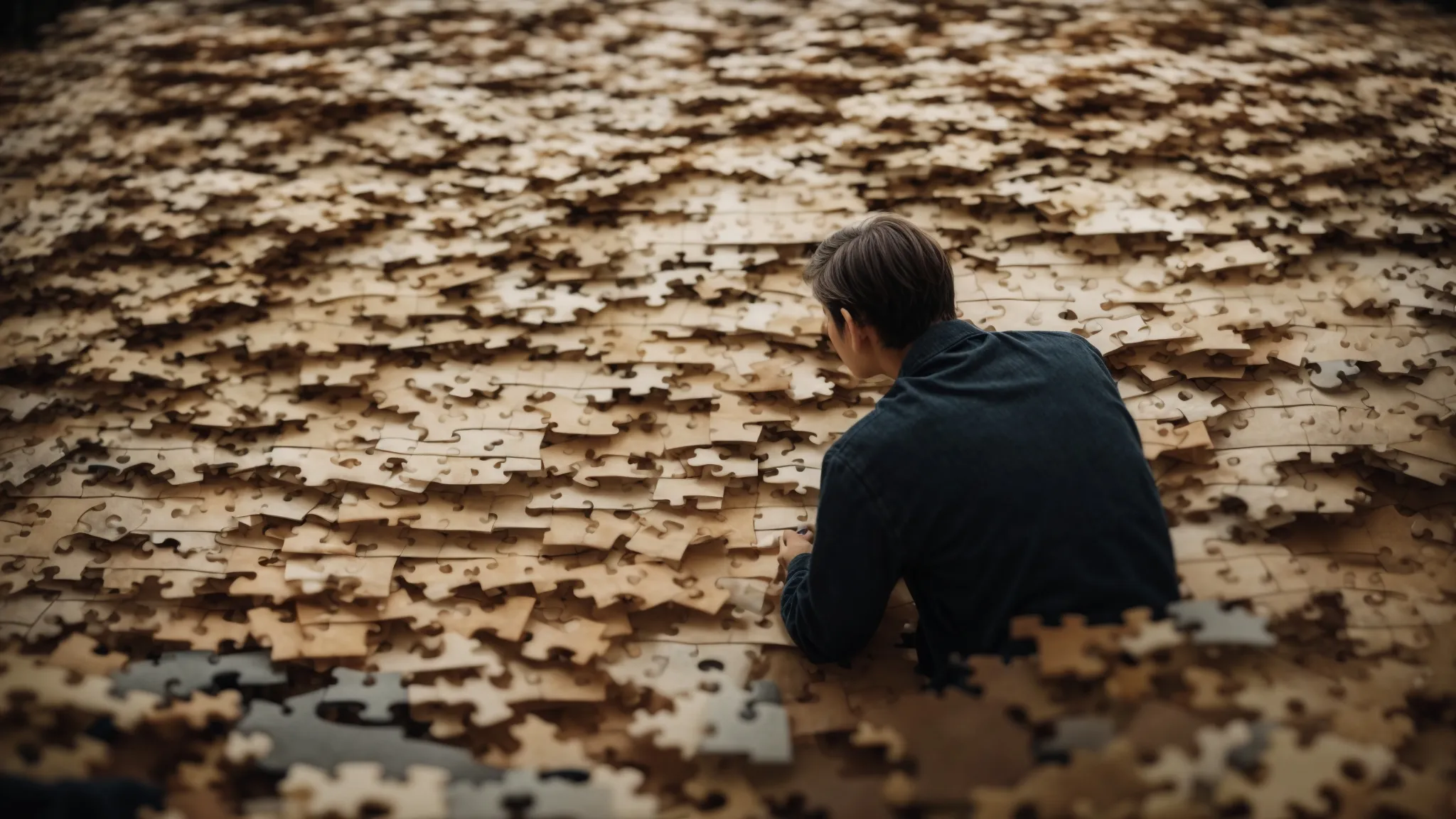
The premise of divide and conquer algorithms hinges on breaking down complex problems into simpler ones, a strategy that has cemented its status in the introduction to algorithms. Consider a binary tree or a search tree, which serve as archetypes for this technique. These structures exemplify how large, intricate sequences can be handled by dividing them into manageable subproblems. A programmer might use a while loop to iteratively address these smaller segments, laying the groundwork for a recursive approach. Each solved piece, akin to a puzzle, brings one closer to the complete image. As recursion whittles down the complexity, the elegance of combine phase comes into play, merging individual solutions into a comprehensive answer. Instances of such methodology abound, demonstrating the algorithm’s effectiveness across various applications.
Breaking Down Complex Problems Into Simpler Ones
The crux of divide and conquer strategies lies in the distillation of unwieldy problems into their constituent elements. Take the fast Fourier transform, for instance, a ubiquitous tool in discrete signal processing that recalibrates the problem of waveform analysis into a series of smaller, conquerable tasks. By partitioning a complex equation into its essential frequencies, these algorithms streamline computational processes where traditional multiplication would falter. Similar principles bolster the efficiency of advanced matrix multiplication algorithms, where the normal approach to multiplication is eschewed in favor of subdividing matrices into quadrants, thereby simplifying the process and hastening computation. Even the pursuit of the median in a numeric array benefits from such tactics, where probability and ordered division guide the algorithm toward a solution with greater speed and less overhead.
Recursively Solving Subproblems
The elegance of divide and conquer is vividly showcased by the Fibonacci sequence, an array of numbers where each element is the sum of the preceding two. When crafting a recursive function to calculate these values, programmers define a base case as a parameter that halts further decomposition of the problem — often when the sequence reaches one or zero. Algorithms like bucket sort, which distribute elements into various ‘buckets’ before sorting them, rely on recursive techniques to attain the expected value with greater fidelity than algorithms like selection sort, which may exhibit inefficiency with larger data sets.
Combining Solutions for the Overall Result
The synthesis phase of divide and conquer algorithms is where fragmented solutions are woven together to construct the final outcome. Whether it involves the rotation of subarrays or the merging of Fourier transform coefficients, this step is pivotal for achieving a harmonious answer. It requires not just precision in mathematics but also an appreciation of technology’s capability to apply concepts like big o notation for optimal performance analysis.
Problem | Division | Conquering Subproblems | Combining with Big O Notation |
---|---|---|---|
Fourier Transforms in Signal Processing | Waveform divided into frequencies | Individual Fourier coefficients calculated | Combined efficiently to reconstruct signal (O(n log n)) |
Array Rotation | Subarrays divided at pivot | Pieces are independently rotated | Merged to complete the array rotation (O(n)) |
Matrix Multiplication | Matrices split into quadrants | Multiplication of smaller matrices | Assembled to form large product matrix (O(n^2.81)) |
Classic Examples Demonstrating the Technique
In the study of computer science, the binary search tree stands as a prime instance of the divide and conquer technique applied in a practical machine context. By storing items in a memory-efficient way, each ‘node’ or bit of data can be compared and sorted efficiently using Java algorithms, which demonstrate the prowess of divide and conquer in organizing vast amounts of information swiftly and with precision.
Technique | Application in Computer Science | Benefit |
---|---|---|
Binary Search Tree | Efficient data sorting and retrieval | Optimizes memory usage and accelerates machine operations |
Time Complexity Analysis of Divide and Conquer
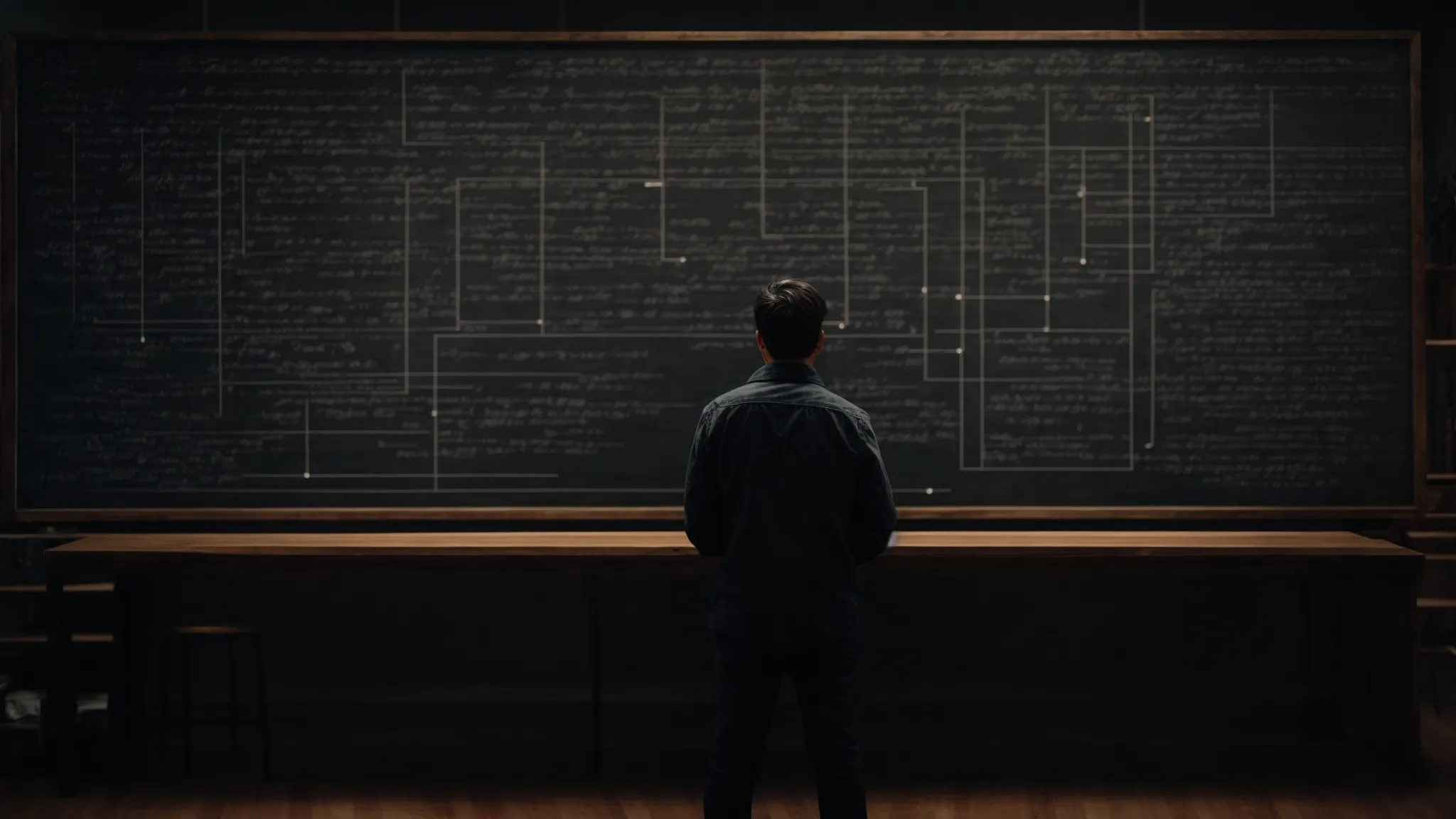
In scrutinizing the prowess of divide and conquer algorithms, time complexity reveals the efficiency of these strategies. Big O Notation provides a definition for quantifying algorithmic performance, essential for comparing sophisticated methods such as recursive matrix multiplication against elementary operations like linear search. By dissecting the time complexity of recursive calls, one can determine the scalability of an approach, particularly relevant for intricate tasks such as the legendary travelling salesman problem. Analyzing classic sorting algorithms like Merge Sort and Quick Sort further emphasizes the differential impacts of algorithmic design on performance. Whether managing the complexity of the tower of Hanoi or executing data sorting, understanding the temporal demands of these tactics is paramount for optimizing problem-solving endeavors.
Understanding Big O Notation
Grasping Big O Notation equips developers with the foresight to assess how solutions scale as data grows, whether one is queuing tasks, employing hash tables for efficient data retrieval, or architecting systems powered by artificial intelligence. For example, a binary search algorithm, optimized for its speed in locating elements, might boast a logarithmic O(log n) performance, a stark contrast to the linear time O(n) seen in simplistic search algorithms. Similarly, a well-designed randomized algorithm can disrupt the predictability of performance outcomes, occasionally delivering enhanced processing times that defy average expectations.
Analyzing the Efficiency of Recursive Calls
The examination of recursive procedures unveils their potent efficiency in algorithms like Quicksort, which partitions data before solving and fuses the sorted arrays with adeptness. In the realm of sorting, Radix Sort operates without comparisons, delegating its efficiency to distribution and collection stages, which, when executed recursively, showcase significant gains in speed for large sets of numerical data. Likewise, in complex operations such as multiplication algorithms and tree traversals, the judicious implementation of recursion in an AVL tree, known for self-balancing, ensures that the performance remains optimized, sidestepping the common pitfalls of unbalanced data structures that exacerbate computational load.
Case Studies: Merge Sort vs. Quick Sort
Amid the various algorithms that embody the divide and conquer approach, Merge Sort and Quick Sort have emerged as quintessential subjects of study for their distinctive handling of sorted arrays. While both algorithms employ recursion extensively, they differ fundamentally in their partition strategies and asymptotic analysis. The elegance of Merge Sort lies in its ability to merge pre-sorted arrays with a consistency reflected in its pseudocode, offering stable sort and a predictable time complexity; conversely, Quick Sort partitions its array around a pivot—selected via diverse heuristics—and can outpace Merge Sort on average, though its worst-case scenario is less favorable.
- The Euclidean algorithm offers insight into recursive problem-solving beyond mere sorting, emphasizing the foundational role of division in algorithmic efficiency.
- Pseudocode for both Merge Sort and Quick Sort illuminates their recursive nature, showing the step-by-step execution that forms the backbone of understanding in computer science education.
- Asymptotic analysis provides a graph-like conceptual landscape where the best, average, and worst-case scenarios can be compared, allowing programmers to make informed choices based on the nature of the dataset involved.
In the continuous quest for efficiency, developers and mathematicians alike turn to the graph of an algorithm’s performance as a map to its practicability, with the euclidean algorithm serving as a testament to the enduring legacy of divide and conquer methods. Thus, when equipping oneself with algorithmic strategies, the understanding gleaned from the comparative study of Merge Sort and Quick Sort becomes a powerful tool in a developer’s repertoire.
Divide and Conquer vs Dynamic Programming
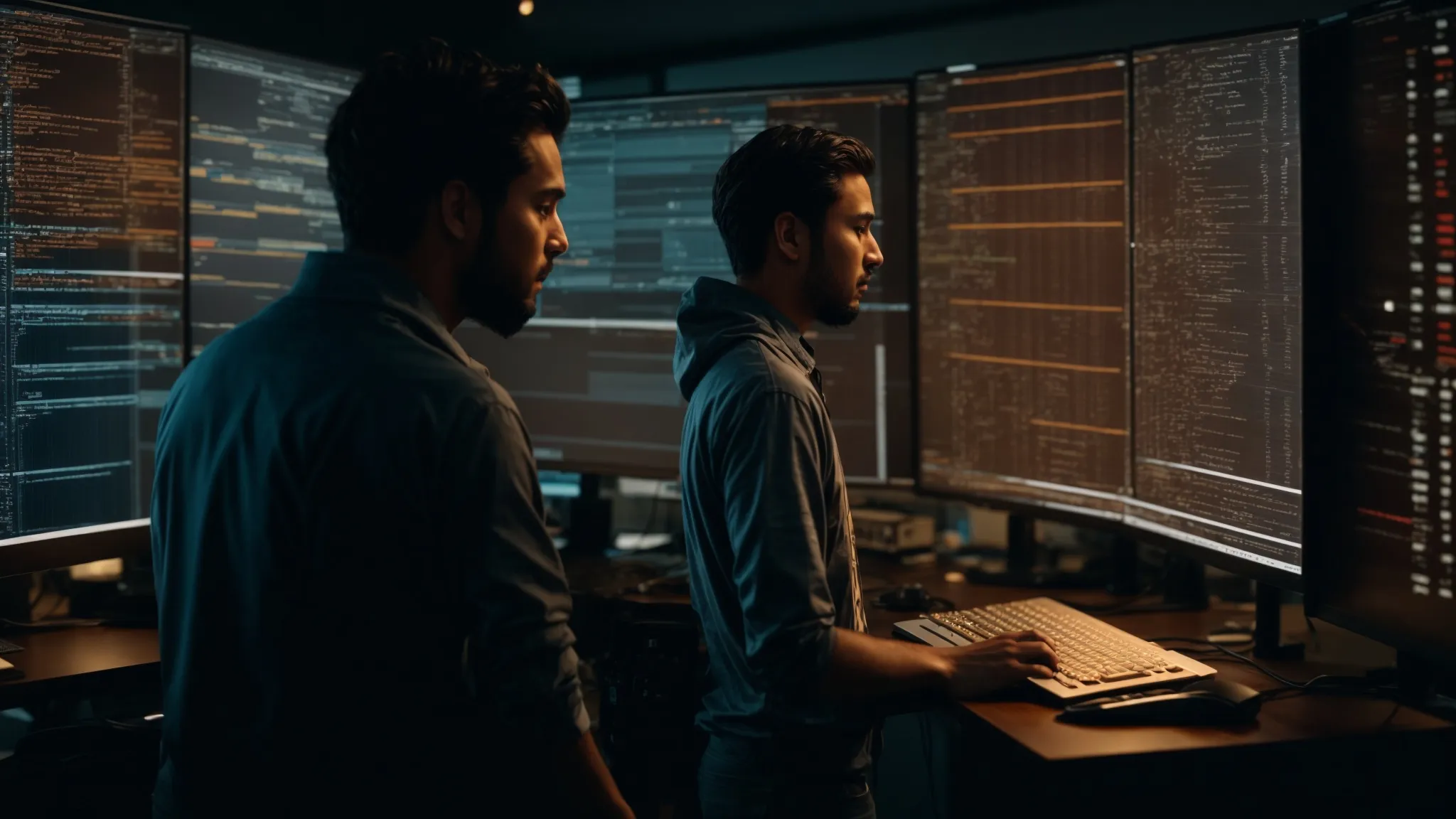
Within the arsenal of algorithmic strategies, the line of distinction between divide and conquer techniques and dynamic programming must be drawn with care, for each offers its unique set of advantages. Developers in the realm of computer science routinely face decisions about which paradigm to employ—be it in scripting complex JavaScript functions, formulating efficient machine learning algorithms, or optimizing the paths of a minimum spanning tree. Identifying key differences in these approaches not only informs the choice of method but also impacts the performance outcomes in solving intricate problems. As practitioners navigate these methods, understanding when to utilize each becomes crucial—dynamic programming may excel in overlapping subproblems, while divide and conquer could offer a more suitable route for distinct, divisible tasks. This critical analysis forms the basis for programmers to execute the most proficient and tailored solutions in their computational endeavors.
Identifying Key Differences in Approach
The interplay between dynamic programming and divide and conquer algorithms is nuanced; dynamic programming hinges on the understanding and application of recurrence relations to break down problems with overlapping subproblems, storing their solutions with meticulous rigor, often through stacks that preserve intermediate states. In contrast, divide and convquer algorithms, such as the Karatsuba algorithm for fast multiplication, decompose problems into non-overlapping subproblems, independently handling each section before merging results, ideal for scenarios like computing the vertex cover of a graph where subproblems are distinct and conquerable in isolation.
Practical Scenarios for Choosing Each Method
When faced with the vast concept of stack overflow in programming, the prudent selection between divide and conquer and dynamic programming hinges on the space complexity and the specific problem at hand. For example, the analysis of algorithms that involve the longest common subsequence is often best approached with dynamic programming due to its adeptness at utilizing past knowledge and reducing redundant calculations, whereas divide and conquer is adept at managing problems that can be broken into independent subproblems without the need for such overlap consideration.
Performance Comparison in Complex Problems
In the landscape of computational science, the practicality of an algorithm when applied to intricate scenarios often determines its adoption. Python, favored for its lucidity and powerful libraries, provides an instrumental angle in comparing the effectiveness of divide and conquer versus dynamic programming. Understanding the nuances of each approach helps developers select the most appropriate data structure or matrix operation to address the complexities of the problem at hand.
- Assess the specifics of the problem to determine if there’s an overlap in subproblems which may necessitate dynamic programming.
- Evaluate the role of Python’s libraries in simplifying the implementation of complex data structures and algorithms.
- Compare time efficiency and resource utilization between methods to gauge the most efficient angle of approach.
Advantages of Divide and Conquer Algorithms
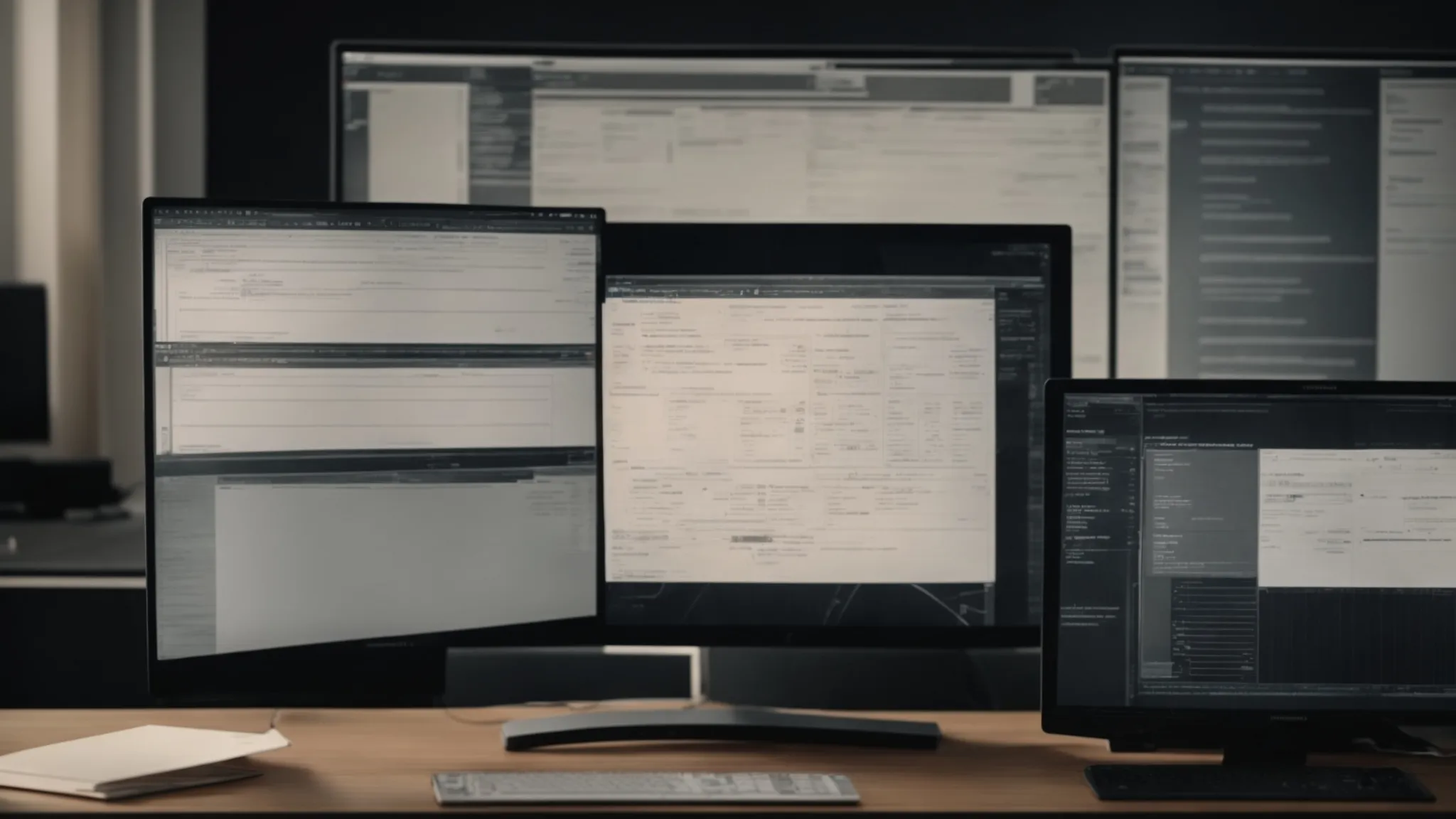
The strategic application of divide and conquer algorithms offers a plethora of benefits, chief among them being a substantial enhancement of computational efficiency. By breaking down a problem into its smallest components, these algorithms decrease space complexity, which allows for faster processing and less memory usage. Programmers can pinpoint errors more swiftly and resolve them with greater accuracy, making the debugging and problem-solving processes much more manageable. Whether determining the maximum and minimum values in a dataset, parsing through a string for pattern matching, or navigating the complexities of backtracking with a pointer, divide and conquer remains versatile, adept at tackling an expansive array of problem types.
Enhancing Computational Efficiency
Divide and conquer algorithms improve computational efficiency by optimizing cache usage, allowing programs to execute faster by reducing the time-consuming operations of data retrieval. Unlike dynamic programming, which extensively stores and recalls solutions to subproblems, as seen in the knapsack problem, divide and conquer algorithms break down a problem and deal with each part independently, minimizing memory access patterns that could otherwise slow down a spanning tree computation. Employing such an algorithm streamlines the process, ensuring computations are both swift and resource-effective.
Simplifying Debugging and Problem Solving
The implementation of divide and conquer strategies, such as matrix chain multiplication and counting sort, has substantially improved the ease of debugging and problem solving. With recursion central to these techniques, programmers can isolate and examine specific portions of code, particularly in complex geometric computations like convex hull determination, enhancing precision in identifying and rectifying anomalies swiftly.
Versatility Across Various Types of Problems
The versatility of divide and conquer algorithms sweeps across computational problem-solving, with applications that range from elementary algorithms like bubble sort to the intricate calculations required for tree data structures. By incorporating concepts such as partition methods and leveraging the analytical power of the master theorem, these algorithms adeptly handle arrays of integers and manage complex recursive relations in a variety of scenarios:
- Bubble sort is enhanced by identifying and implementing effective partition strategies, ensuring improved performance on sorting integers.
- Tree structures benefit from divide and conquer methods to optimize traversal and manipulation, adapting the approach to different variants of trees.
- The master theorem serves as a critical tool for analyzing the time complexity of recursive algorithms, providing a clear framework for their application.
Implementing Divide and Conquer in Real-World Applications
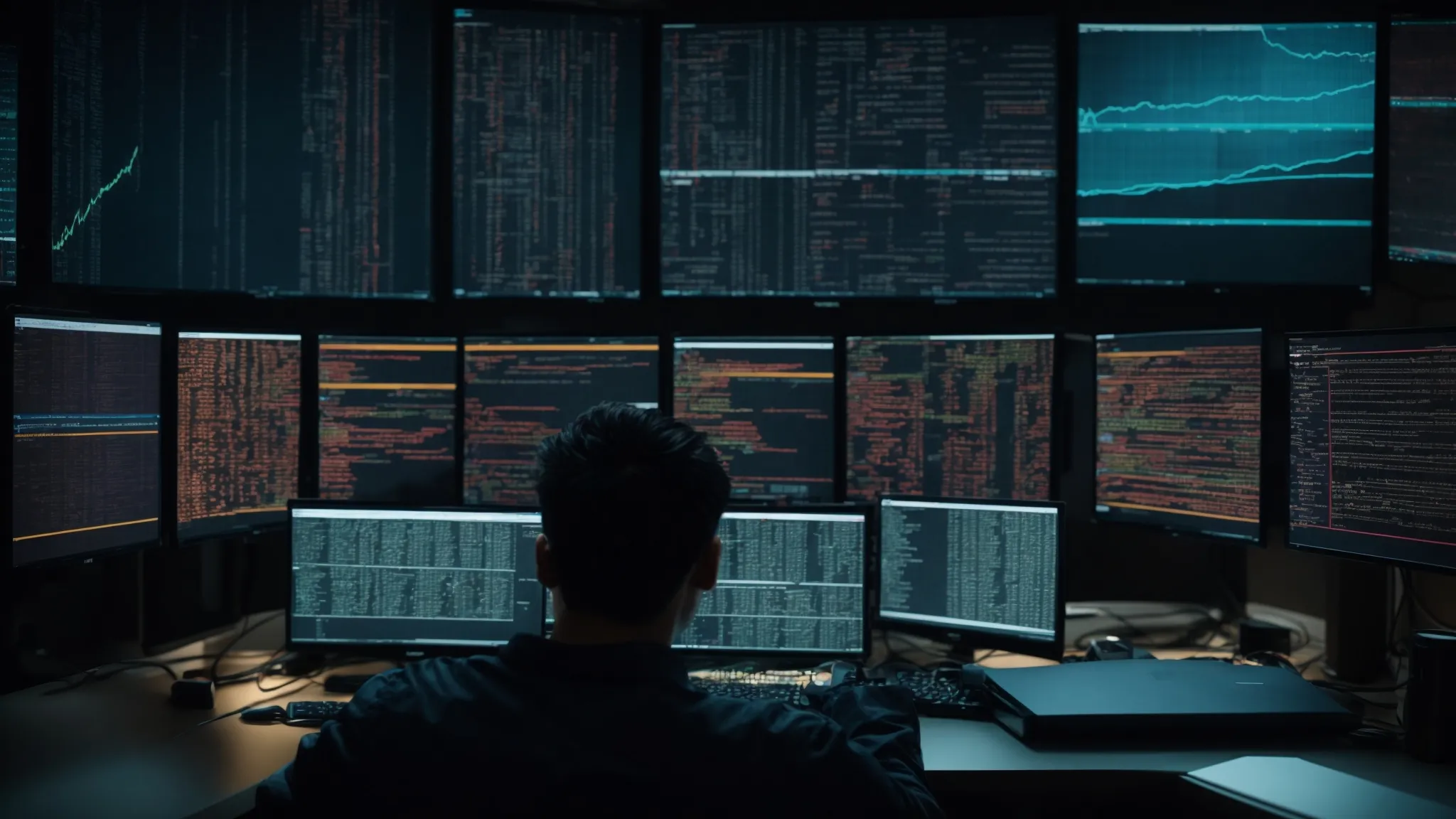
In the quest to navigate the realm of algorithms, practicality reigns supreme, with divide and conquer standing as a bastion of efficiency. Real-world challenges often manifest as elaborate mazes, dense with complexity, in domains as varied as data sorting and cryptography. Amid such intricacies, implementing divide and conquer tactics not only illuminates the path to solution but also sharpens the user’s experience. Whether it is seamlessly sifting through linked lists to identify a number, dissecting intricate shapes in computational geometry, or safeguarding sensitive information, these algorithms offer robust foundations upon which feedback mechanisms propel precision and accuracy. From speeding up searches in vast databases to deciphering the complexities of data analysis, divide and conquer enables an elegant orchestration of steps that combat the multifaceted challenges encountered in technology and computation.
Application in Sorting and Searching Algorithms
In the realm of algorithms, sorting and searching functions stand as critical challenges that continually benefit from the learning and application of divide and conquer strategies. Merge Sort, in particular, showcases the power of this approach by dividing a dataset into smaller arrays to be sorted and then merging them into a single, ordered sequence. Similarly, Tower of Hanoi puzzles represent the strategic essence of divide and conquer as a recursive learning model, where the solution requires an algorithm to move disks between pegs, abiding by specific rules: a paradigm exercise for understanding recursive functions in sorting algorithms.
Algorithm | Division | Conquering Technique | Learning Outcome |
---|---|---|---|
Merge Sort | Dataset split into smaller arrays | Sort and merge phases | Understanding of recursive sorting mechanisms |
Tower of Hanoi | Disks divided among three pegs | Recursive disk movement respecting game rules | Comprehension of complex recursive functions |
Utilization in Computational Geometry
In computational geometry, divide and conquer algorithms are applied to cope with complex geometrical structures and problems with efficiency. By breaking down geometrical data into more manageable subproblems, such as partitioning an array of points for the closest pair problem, these algorithms can significantly accelerate the calculation process. Employing heaps for space optimization, utilizing parallel computing and multiprocessing techniques for handling extensive geometrical computations, or implementing a greedy algorithm for a convex hull problem, divide and conquer strategies adapt seamlessly to the demands of computational geometry, offering solutions that are both precise and swift.
Geometrical Problem | Division Strategy | Conquering Mechanism | Tools Used |
---|---|---|---|
Closest Pair of Points | Divide array of points | Compare distances in subarrays | Heap for space optimization |
Convex Hull | Partition points set | Separate hulls combined | Greedy algorithm |
Large Geometrical Structures | Break down complex shape | Process segments concurrently | Parallel computing |
Role in Cryptography and Data Analysis
In the domain of cryptography, divide and conquer principles refine the efficiency of encrypting and decrypting data, just as the elegant iterations of an insertion sort optimize the organization of information. Huffman coding, a widely recognized algorithm for data compression, capitalizes on the tactic of breaking down frequencies into a binary tree structure, ensuring each step leads incrementally towards the final solution. By dissecting complex encryption tasks, these algorithms accelerate the processing of secure communications, enabling rapid and secure data transmission across digital platforms.
- Refining cryptographic processes by dividing encryption tasks into simpler units.
- Optimizing data organization through iterative techniques akin to insertion sort.
- Employing Huffman coding for efficient data compression by constructing binary trees.
Mastering Divide and Conquer Through Examples
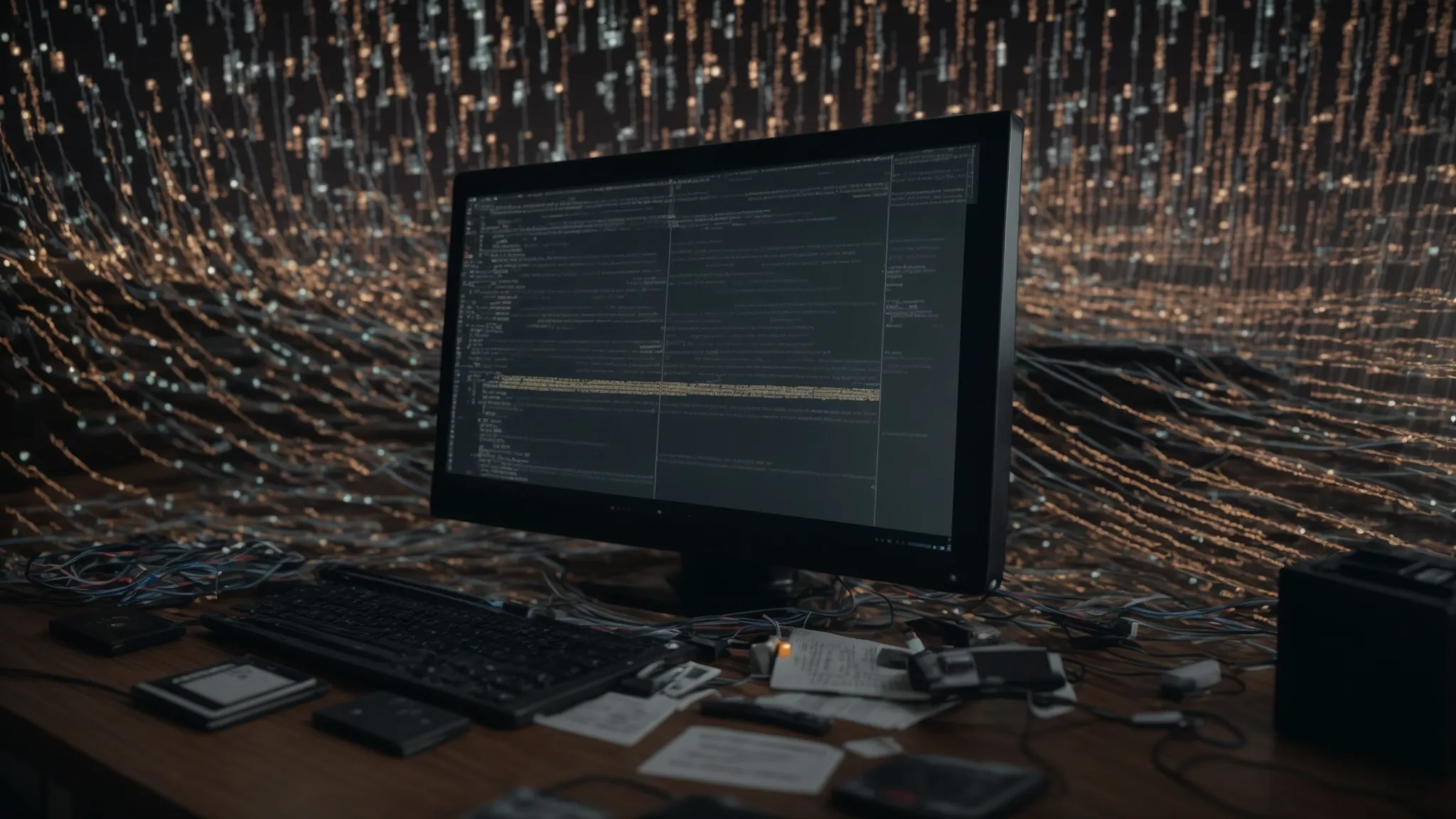
The journey toward proficiency in divide and conquer algorithms is enhanced by hands-on exposure to classic examples that illuminate their power and efficiency in sorting and organizing data. A detailed exploration into the workings of Merge Sort, from the initial division to the final merge, offers practitioners a foundational understanding of recursive sorting techniques. Equally instructional is the dissection of Quick Sort, which reveals the nuances of pivot selection and partition-based sorting operations. Beyond these, the realm of sophisticated computational tasks opens up with the introduction of multithreaded algorithms that harness the divide and conquer philosophy, efficiently tackling problems by distributing the workload across multiple processing threads. Through these practical illustrations, knowledge of the intricate relationships between algorithms and the handling of complex data is deepened, equipping developers with the dexterity to solve an extensive array of computational challenges.
Step-by-Step Guide to Merge Sort
A Merge Sort algorithm embarks on its process by recursively splitting the input array into halves until solitary elements remain. Following the division, it embarks on a merging journey where each piece, now sorted within itself, is seamlessly combined with its counterpart, ensuring order is meticulously maintained. The final act is a symphony of merges, culminating in a completely sorted array—a performance where both the simplicity of the problem breakdown and the harmony of the merging process define Merge Sort’s elegance and power.
Quick Sort Explained
Quick Sort operates on the principle of choosing a ‘pivot’ element from the array and partitioning the other elements into two subarrays, according to whether they are less than or greater than the pivot. The magic happens with its clever use of recursion to sort the subarrays, a process that continues until no more division is possible, resulting in a fully sorted collection. Its performance hinges on the choice of pivot, which if chosen wisely, allows Quick Sort to achieve average time complexities that make it highly favored for sorting tasks.
Multithreaded Algorithms Using Divide and Conquer
Multithreaded algorithms embody the divide and conquer creed by parallelizing the processing of subproblems, which can dramatically accelerate computation times. By leveraging the power of concurrency, these algorithms decompose the problem space, enabling individual threads to tackle complex calculations simultaneously with greater efficiency. This approach not only optimizes the use of multi-core processors but also minimizes idle time, facilitating more expeditious and scalable solutions to computationally intensive tasks.
Conclusion
Mastering divide and conquer algorithms equips programmers with a robust framework for decomposing complex problems into simpler, solvable components. These algorithms enhance computational efficiency, streamline debugging, and offer adaptability across a vast range of challenges, from sorting data to cryptography. By learning through practical examples like Merge Sort and Quick Sort, developers deepen their understanding of recursive techniques and improve their problem-solving capabilities. Embracing this methodology is fundamental for crafting effective, scalable, and resource-efficient solutions in the field of computer science.